Lesson contents
The dropdown is one of the easiest BS components, so it's a good place to start.
What is a dropdown?
Say you're writing a choose-your-own-adventure game. One of these things:
Here's what your page might look like:
The choices are a
tags. Each one jumps to a different page. You can try it.
Making one
We'll:
- Find example HTML
- Paste it into a page
- Change it to do what we want
This is the process for all the components.
Remember, use the sample BS template given earlier.
Find some template HTML
The best place is on the official BS site. There are other templates on the web, lots of them. If you use one, make sure it's for BS 5.
I always use templates from the official site, just to be safe.
Take a look at the official dropdown page. It has many examples.
Here's what we want.
It has two pieces:
- The trigger button that shows the options list
- The options list.
Here's the code from the BS docs:
- <div class="dropdown">
- <button class="btn btn-secondary dropdown-toggle" type="button" data-bs-toggle="dropdown" aria-expanded="false">
- Dropdown button
- </button>
- <ul class="dropdown-menu">
- <li><a class="dropdown-item" href="#">Action</a></li>
- <li><a class="dropdown-item" href="#">Another action</a></li>
- <li><a class="dropdown-item" href="#">Something else here</a></li>
- </ul>
- </div>
Lines 1 and 10 wrap the component. Lines 2–4 make the trigger button. Lines 5–9 make the options list.
You can change btn-secondary
on line 2 to change how the button looks. btn-secondary
makes a button like this:
If you use btn-primary
:
There are nine different styles, listed on the buttons page.
Here's the options section again:
- <ul class="dropdown-menu">
- <li><a class="dropdown-item" href="#">Action</a></li>
- <li><a class="dropdown-item" href="#">Another action</a></li>
- <li><a class="dropdown-item" href="#">Something else here</a></li>
- </ul>
It's just a list of links to other pages. Replace each #
with a URL.
Paste the code
The next step is to paste the code into our BS template. Here's the template again:
- <!doctype html>
- <html lang="en">
- <head>
- <meta charset="utf-8">
- <meta name="viewport" content="width=device-width, initial-scale=1">
- <title>Dropdown</title>
- <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-gH2yIJqKdNHPEq0n4Mqa/HGKIhSkIHeL5AyhkYV8i59U5AR6csBvApHHNl/vI1Bx" crossorigin="anonymous">
- </head>
- <body>
- <div class="container">
- <div class="row">
- <div class="col">
- My code
- </div>
- </div>
- </div>
- <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/js/bootstrap.bundle.min.js" integrity="sha384-A3rJD856KowSb7dwlZdYEkO39Gagi7vIsF0jrRAoQmDKKtQBHUuLZ9AsSv4jD4Xa" crossorigin="anonymous"></script>
- </body>
- </html>
You know how to paste. Copy-and-paste is good.
Customize
Here are the steps again:
- Find example HTML >>Done<<
- Paste it into our page >>Done<<
- Change it to do what we want >>Now<<
Here's what I wrote.
- <h1>Doggos of Doom</h1>
- <p>
- You see a cute doggo.
- </p>
- <p>
- <img src="dog.png" alt="Doggo" class="img-fluid">
- </p>
- <div class="dropdown">
- <button class="btn btn-primary dropdown-toggle" type="button" data-bs-toggle="dropdown" aria-expanded="false">
- What do you do?
- </button>
- <ul class="dropdown-menu">
- <li><a class="dropdown-item" href="pet-doggo.html">Pet the doggo</a></li>
- <li><a class="dropdown-item" href="play-with-doggo.html">Play with the doggo</a></li>
- </ul>
- </div>
You should be able to match up the tags with the output:
Here's the component's code again:
- <div class="dropdown">
- <button class="btn btn-primary dropdown-toggle" type="button" data-bs-toggle="dropdown" aria-expanded="false">
- What do you do?
- </button>
- <ul class="dropdown-menu">
- <li><a class="dropdown-item" href="pet-doggo.html">Pet the doggo</a></li>
- <li><a class="dropdown-item" href="play-with-doggo.html">Play with the doggo</a></li>
- </ul>
- </div>
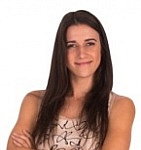
Georgina
What's that data-bs-toggle
in line 2?
That's used by the JavaScript code that makes the dropdown work. Don't worry about what it means. Just be certain it's there. If it's not, the code won't work.
Same with aria-expanded
on the same line. Just make sure it's there.
Using BS components is like that. You paste code, without knowing exactly what each piece does. That's OK, but you might have to mess around to get things to work. There are many examples in the docs to help you. Ask me (Kieran) if you get stuck. Gather.Town is the best place.
Checking for JavaScript errors
Things like dropdowns and navbars rely on code in the programming language JavaScript. The code is inserted into your pages at the bottom of the BS template. Don't worry, you don't need to know how it works. You can ignore it most of the time.
However, sometimes you get JS errors if your code isn't correct. The error messages can help you figure out what's wrong.
To see them, use the browser's dev tools we talked about earlier. Press F12 (on a PC). Click the console tab:
You'll see errors there.
Not every problem creates error messages, unfortunately. Life would be easier if they did.
Exercise
Campus adventure
Write a short choose-your-own-adenture game. The first page should start with, "I was walking on campus, when..."
Have at least four pages.
Use Bootstrap.
Submit the URL of your solution. The usual coding standards apply.
Up next
Let's talk about navbars.