Lesson contents
Warning!
This part of the course was updated recently. It was about Bootstrap version 4. Now it's about BS 5.
If you find old content about BS 4, please tell Kieran at mathieso at oakland.edu.
Making a Bootstrap page
Twitter made Bootstrap. It's a bunch of templates, along with stylesheets, and other things. We can copy the templates, and change them as we want. Bootstrap has been around for a while. It's on version 5, and it's mighty.
It's free. Free is good.
Here's a basic template for a page that uses Bootstrap. Use this template for this part of the course, not the template you've been using so far.
- <!doctype html>
- <html lang="en">
- <head>
- <meta charset="utf-8">
- <meta name="viewport" content="width=device-width, initial-scale=1">
- <title>Bootstrap template</title>
- <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-gH2yIJqKdNHPEq0n4Mqa/HGKIhSkIHeL5AyhkYV8i59U5AR6csBvApHHNl/vI1Bx" crossorigin="anonymous">
- </head>
- <body>
- <div class="container">
- <div class="row">
- <div class="col">
- <h1>Doggos</h1>
- <p>Doggos are the <em>best!</em></p>
- </div>
- </div>
- </div>
- <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/js/bootstrap.bundle.min.js" integrity="sha384-A3rJD856KowSb7dwlZdYEkO39Gagi7vIsF0jrRAoQmDKKtQBHUuLZ9AsSv4jD4Xa" crossorigin="anonymous"></script>
- </body>
- </html>
Use this template for your code.
You can see the page in action. Here's what it looks like:
The fonts are set nicely, and the content has a left margin. Not too much yet, but as we add stuff, it'll get pretty fancy.
The template bits
There are two main parts of the template. First, there's this in the head
:
- <meta charset="utf-8">
- <meta name="viewport" content="width=device-width, initial-scale=1">
- <title>Bootstrap template</title>
- <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-gH2yIJqKdNHPEq0n4Mqa/HGKIhSkIHeL5AyhkYV8i59U5AR6csBvApHHNl/vI1Bx" crossorigin="anonymous">
The last line loads a CSS file.
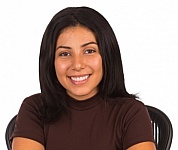
Adela
What's the integrity
thing? And crossorigin
?
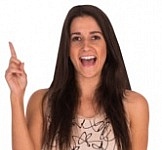
Georgina
Ooo! I know!
What is it?
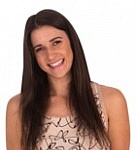
Georgina
It's security stuff. integrity
is like a unique number for the CSS file. The browser downloads what the link
tag points to, and checks that the integrity code matches what it downloaded. Stops someone sneaking an Evil File in.
crossorigin
has to do with one site grabbing a file from another. That has to be allowed, since the CSS file is coming from a CDN, not our website.
Good! That's what those things are.
Copy the template as-is, and you won't have problems.
The Bootstrap CSS file
The CSS file is loaded by the link
tag.
- <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-gH2yIJqKdNHPEq0n4Mqa/HGKIhSkIHeL5AyhkYV8i59U5AR6csBvApHHNl/vI1Bx" crossorigin="anonymous">
Take a look at the URL in the href
. Rather than loading the CSS file from our website, we get the file from a CDN, or content delivery network. CDNs are fast servers located around the world, that serve up commonly used files quickly.
You can put the Bootstrap CSS file on your server if you'd like. Many people do that. But you don't have (I don't).
Once you load bootstrap.min.css
(from a CDN, or your server), you can use the classes defined in the file, as if you had made them yourself. For example, you can make a link look like a button:
- <a class="btn btn-primary" href="https://google.com">Google</a>
The classes btn
and btn-primary
are defined in bootstrap.min.css
. You don't define them yourself. Here's what the link would look like:
As long as you know what classes Bootstrap gives you, you just use them, and get Cool Stuff.
The Bootstrap JavaScript file
Just before the body
tag, there's:
- <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/js/bootstrap.bundle.min.js" integrity="sha384-A3rJD856KowSb7dwlZdYEkO39Gagi7vIsF0jrRAoQmDKKtQBHUuLZ9AsSv4jD4Xa" crossorigin="anonymous"></script>
This loads a JavaScript file from a CDN. JavaScript is a programming language. You won't be learning JavaScript in this course. Just include the file. Some components you'll learn about later need it.
The template's body
Here's the body
of the template:
- <body>
- <div class="container">
- <div class="row">
- <div class="col">
- <h1>Doggos</h1>
- <p>Doggos are the <em>best</em>!</p>
- </div>
- </div>
- </div>
- ...
- </body>
Replace the stuff in the col
div, and you have a Bootstrap page.
Bootstrap has a w00fy grid system, that lets you make cool page layouts. Grids are made of rows and columns, all wrapped up in a container. You can see the classes container
, row
, and col
, that make the grid system work.
We'll talk about the grid system later. It's one of the best parts of Bootstrap.
Exercise
Doggos like Bootstrap
Doggos love Bootstrap. It makes their web lives sooo much easier.
Make a page that looks something like this:
Use the basic Bootstrap template in the Get Bootstrappy lesson. Use whatever animal and text you like. Make sure the link goes to the official Bootstrap site, whatever that is.
Up next
Let's use a few basic Bootstrap classes to make some Cool Stuff.